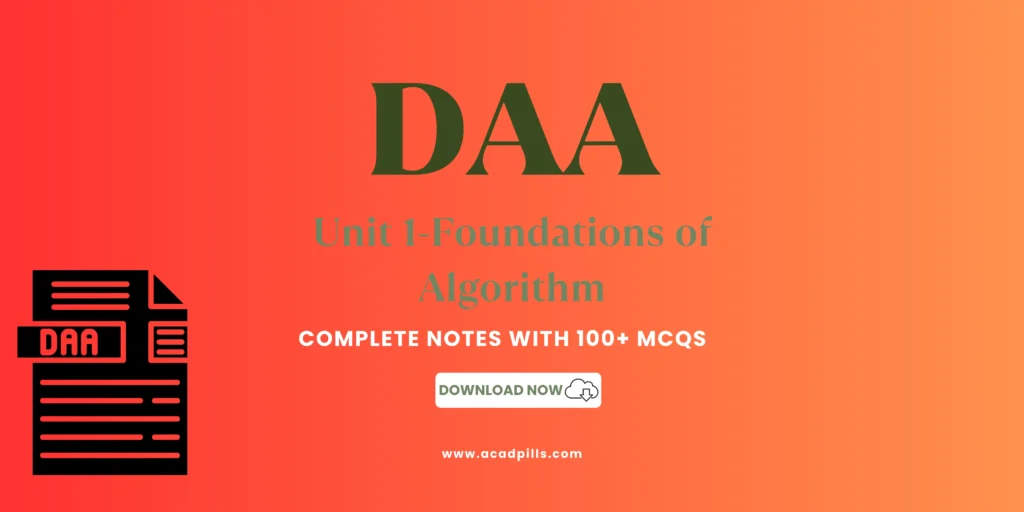
Design and Analysis of Algorithms (DAA) is a core subject for B.Tech Computer Science and Engineering (CSE) students, focusing on designing efficient algorithms to solve various computational problems. It involves developing strategies for problem-solving and analyzing the algorithms’ efficiency.
Foundations of Algorithms
Algorithm: An algorithm is a sequence of steps or instructions designed to solve a specific problem. It’s a precisely defined procedure for obtaining answers, emphasizing the “how” rather than just the “what”. Key requirements of an algorithm include:
- Finiteness: Must terminate after a finite number of steps.
- Definiteness: Each step is rigorously and unambiguously specified.
- Input: Clearly specified valid inputs.
- Output: Clearly specified and expected output.
Fundamentals of Algorithmic Problem Solving: This involves understanding the problem thoroughly before designing a solution. Key steps include:
- Understanding the Problem: Read the problem description carefully.
- Ascertaining Computational Devices Capabilities: Understanding whether to implement an exact or approximate solution, and the data structures that are appropriate for the algorithm.
- Choosing Exact vs. Approximate Solution: Decide whether an exact solution is needed or if an approximate solution is sufficient.
- Algorithm Design Techniques: Utilizing appropriate algorithm design techniques.
- Proving Correctness: Ensuring the algorithm produces the correct output for all valid inputs.
- Analyzing Algorithms: Analyzing the time and space complexity of the algorithm.
- Coding an Algorithm: Implementing the algorithm in a suitable programming language.
Basic Algorithm Design Techniques: Several fundamental techniques are used to design algorithms:
- Divide and Conquer: Divide the problem into smaller subproblems, solve them recursively, and combine the solutions.
- Greedy Method: Make locally optimal choices at each step with the hope of finding a global optimum.
- Dynamic Programming: Solve overlapping subproblems by storing their solutions to avoid redundant computations.
- Backtracking: Systematically search for a solution by trying different options and undoing choices when they lead to a dead end.
- Branch and Bound: Similar to backtracking but uses bounding functions to prune the search space.
Analyzing Algorithms: Analyzing algorithms involves evaluating their performance in terms of time and space complexity.
- Time Complexity: The amount of time required by an algorithm to complete.
- Space Complexity: The amount of memory space required by an algorithm.
Fundamental Data Structures
Linear Data Structures: Data structures where elements are arranged in a sequential manner.
- Arrays: Collection of elements of the same type, accessed by index.
- Linked Lists: Collection of nodes, each containing data and a reference to the next node.
- Stacks: Follows the Last-In-First-Out (LIFO) principle.
- Queues: Follows the First-In-First-Out (FIFO) principle.
Graphs and Trees: Non-linear data structures representing relationships between data elements.
- Graphs: Consist of nodes (vertices) and connections between nodes (edges).
- Trees: Hierarchical data structures with a root node and child nodes.
Fundamentals of the Analysis of Algorithm Efficiency
Measuring Input Size: The input size significantly affects an algorithm’s performance. It’s the number of elements in the input that affects the running time.
Units for Measuring Running Time: Running time is measured in terms of the number of basic operations performed.
Order of Growth: Describes how the time or space complexity grows as the input size increases.
Worst-Case, Best-Case, and Average-Case Efficiencies:
- Worst-Case Efficiency: The maximum number of steps an algorithm takes for any input of a given size.
- Best-Case Efficiency: The minimum number of steps an algorithm takes for any input of a given size.
- Average-Case Efficiency: The average number of steps an algorithm takes over all possible inputs of a given size.
Asymptotic Notations and Basic Efficiency Classes
Asymptotic Notations: Mathematical notations used to describe the limiting behavior of a function when the argument tends towards a particular value or infinity.
- Big-O Notation (O): Describes the upper bound of an algorithm’s growth rate.
O(g(n))
represents the set of functions that grow no faster thang(n)
, asymptotically. - Big-Omega Notation (Ω): Describes the lower bound of an algorithm’s growth rate.
Ω(g(n))
represents the set of functions that grow at least as fast asg(n)
, asymptotically. - Big-Theta Notation (Θ): Describes the tight bound of an algorithm’s growth rate.
Θ(g(n))
represents the set of functions that grow at the same rate asg(n)
, asymptotically.
Useful Property Involving the Asymptotic Notations:
- If
f(n)
isO(g(n))
andf(n)
isΩ(g(n))
, thenf(n)
isΘ(g(n))
.
Using Limits for Comparing Orders of Growth: Limits can be used to compare the growth rates of two functions.
- If
lim (n→∞) f(n)/g(n) = 0
, thenf(n)
grows slower thang(n)
. - If
lim (n→∞) f(n)/g(n) = c
(where0 < c < ∞
), thenf(n)
andg(n)
grow at the same rate. - If
lim (n→∞) f(n)/g(n) = ∞
, thenf(n)
grows faster thang(n)
.
DAA -Foundations of Algorithm MCQs with answer
Don’t click on the REVEAL BUTTON directly. First, try to answer the question by selecting the correct answer. If you’re unsure of the answer, you can check it by clicking the REVEAL BUTTON. However, you can also see the answer after attempting the question.